2. 1. Variable
2.1 Variable : In a program, a variable is a named item, such as x or numPeople, used to hold a value.
2.2 Variable Assignment, Variable Declaration, : An assignment assigns a variable with a value, such as x = 5. That assignment means x is assigned with 5, and x keeps that value during subsequent assignments, until x is assigned again.
An assignment's left side must be a variable. The right side can be an expression, so an assignment may be x = 5, y = x, or z = x + 2. The 5, x, and x + 2 are each an expression that evaluates to a value.
In programming, = is an assignment of a left-side variable with a right-side value. = is NOT equality as in mathematics. Thus, x = 5 is read as "x is assigned with 5", and not as "x equals 5". When one sees x = 5, one might think of a value being put into a box.
Increasing a variable's value by 1, as in x = x + 1, is common, and known as incrementing the variable.
2.2 Variable declarations(int)
A variable declaration is a statement that declares a new variable, specifying the variable's name and type. Ex: int userAge; declares a new variable named userAge that can hold an integer value. The compiler allocates a memory location for userAge capable of storing an integer. Ex: In the animation below, the compiler allocated userAge to memory location 97, which is known as the variable's address. The choice of 97 is arbitrary and irrelevant to the programmer, but the idea that a variable corresponds to a memory location is important to understand.
When a statement that assigns a variable with a value executes, the processor writes the value into the variable's memory location. Likewise, reading a variable's value reads the value from the variable's memory location. The programmer must declare a variable before any statement that assigns or reads the variable, so that the variable's memory location is known.
Note: Capitalization matters, so MyNumber is not the same as myNumber.
Assignment statements
An assignment statement assigns the variable on the left-side of the = with the current value of the right-side expression. Ex: numApples = 8; assigns numApples with the value of the right-side expression (in this case 8). assign
An expression may be a number like 80, a variable name like numApples, or a simple calculation like numApples + 1. Simple calculations can involve standard math operators like +, -, and *, and parentheses as in 2 * (numApples - 1). An integer like 80 appearing in an expression is known as an integer literal.
Initializing variables
Although not required, an integer variable is often assigned an initial value when declared. Ex: int maxScore = 100; declares an int variable named maxScore with an initial value of 100.
Assignment statement with same variable on both sides
Commonly, a variable appears on both the right and left side of the = operator. Ex: If numItems is 5, after numItems = numItems + 1; executes, numItems will be 6. The statement reads the value of numItems (5), adds 1, and assigns numItems with the result of 6, which replaces the value previously held in numItems.
2.3 Identifier
Rules for identifiers
A name created by a programmer for an item like a variable or method is called an identifier. An identifier must:
- be a sequence of letters (a-z, A-Z), underscore (_), dollar signs ($), and digits (0-9)
- start with a letter, underscore, or dollar sign
Identifiers are case sensitive, meaning upper and lower case letters differ. So numCats and NumCats are different.
A reserved word is a word that is part of the language, like int, short, or double. A reserved word is also known as a keyword. A programmer cannot use a reserved word as an identifier. Many language editors will automatically color a program's reserved words. A list of reserved words appears at the end of this section.
Style guidelines for identifiers
While various (crazy-looking) identifiers may be valid, programmers may follow identifier naming conventions (style) defined by their company, team, teacher, etc. Two common conventions for naming variables are:
- Camel case: Lower camel case abuts multiple words, capitalizing each word except the first, as in numApples or peopleOnBus.
- Underscore separated: Words are lowercase and separated by an underscore, as in num_apples or people_on_bus.
Neither convention is better. The key is to be consistent so code is easier to read and maintain.
Good practice is to create meaningful identifier names that self-describe an item's purpose. Good practice minimizes use of abbreviations in identifiers except for well-known ones like num in numPassengers. Programmers must strive to find a balance. Abbreviations make programs harder to read and can lead to confusion. Long variable names, such as averageAgeOfUclaGraduateStudent may be meaningful, but can make subsequent statements too long and thus hard to read.
Lower camel case is used for variable naming, which is a common Java naming convention. This material strives to follow another good practice of using two or more words per variable such as numStudents rather than just students, to provide meaningfulness, to make variables more recognizable when variable names appear in writing like in this text or in a comment, and to reduce conflicts with reserved words or other already-defined identifiers.
abstract assert boolean break byte case catch char class const continue default do double else enum extends |
final finally float for goto if implements import instanceof int interface long native new package private protected |
public return short static strictfp super switch synchronized this throw throws transient try void volatile while |
2.4 Arithmetic expressions (general)
Basics
An expression is any individual item or combination of items, like variables, literals, operators, and parentheses, that evaluates to a value, like 2 * (x + 1). A common place where expressions are used is on the right side of an assignment statement, as in y = 2 * (x + 1).
A literal is a specific value in code like 2. An operator is a symbol that performs a built-in calculation, like +, which performs addition. Common programming operators are shown below.
Table 2.4.1: Arithmetic operators.
+ | The addition operator is +, as in x + y. |
- | The subtraction operator is -, as in x - y. Also, the - operator is for negation, as in -x + y, or x + -y. |
* | The multiplication operator is *, as in x * y. |
/ | The division operator is /, as in x / y. |
Evaluation of expressions
An expression evaluates to a value, which replaces the expression. Ex: If x is 5, then x + 1 evaluates to 6, and y = x + 1 assigns y with 6.
An expression is evaluated using the order of standard mathematics, such order known in programming as precedence rules, listed below.
Table 2.4.2: Precedence rules for arithmetic operators.
Operator/Convention | Description | Explanation |
( ) | Items within parentheses are evaluated first | In 2 * (x + 1), the x + 1 is evaluated first, with the result then multiplied by 2. |
unary - | - used for negation (unary minus) is next | In 2 * -x, the -x is computed first, with the result then multiplied by 2. |
* / % | Next to be evaluated are *, /, and %, having equal precedence. | (% is discussed elsewhere) |
+ - | Finally come + and - with equal precedence. | In y = 3 + 2 * x, the 2 * x is evaluated first, with the result then added to 3, because * has higher precedence than +. Spacing doesn't matter: y = 3+2 * x would still evaluate 2 * x first. |
left-to-right | If more than one operator of equal precedence could be evaluated, evaluation occurs left to right. | In y = x * 2 / 3, the x * 2 is first evaluated, with the result then divided by 3. |
A common error is to omit parentheses and assume a different order of evaluation than actually occurs, leading to a bug. Ex: If x is 3, then 5 * x+1 might appear to evaluate as 5 * (3+1) or 20, but actually evaluates as (5 * 3) + 1 or 16 (spacing doesn't matter). Good practice is to use parentheses to make order of evaluation explicit, rather than relying on precedence rules, as in: y = (m * x) + b, unless order doesn't matter as in x + y + z.
2.5 Arithmetic expressions (int)
Style: Single space around operators
A good practice is to include a single space around operators for readability, as in numItems + 2, rather than numItems+2. An exception is minus used as negative, as in: xCoord = -yCoord. Minus (-) used as negative is known as unary minus.
Compound operators
Special operators called compound operators provide a shorthand way to update a variable, such as userAge += 1 being shorthand for userAge = userAge + 1. Other compound operators include -=, *=, /=, and %=.
In the above example, a userAge value of 57 or greater may yield an incorrect output for totalHeartbeats. The reason is that an int variable can typically only hold values up to about 2 billion; trying to store larger values results in "overflow". Other sections discuss overflow as well as other data types that can hold larger values.
2.6 Floating-point (double) variables
A floating-point number is a real number containing a decimal point that can appear anywhere (or "float") in the number. Ex: 98.6, 0.0001, or -55.667. A double variable stores a floating-point number. Ex: double milesTravel; declares a double variable.
A floating-point literal is a number with a fractional part(분수), even if the fraction is 0, as in 1.0, 0.0, or 99.573. Good practice is to always have a digit before the decimal point, as in 0.5, since .5 might mistakenly be viewed as 5.
Scanner's nextDouble() method reads a floating-point value from input. Ex: currentTemp = scnr.nextDouble(); reads a floating-point value from the input and assigns currentTemp with that value.
Scientific notation
Very large and very small floating-point values may be printed using scientific notation. Ex: If a floating variable holds the value 299792458.0 (the speed of light in m/s), the value will be printed as 2.99792458E8.
Choosing a variable type (double vs. int)
A programmer should choose a variable's type based on the type of value held.
- Integer variables are typically used for values that are counted, like 42 cars, 10 pizzas, or -95 days.
- Floating-point variables are typically used for measurements, like 98.6 degrees, 0.00001 meters, or -55.667 degrees.
- Floating-point variables are also used when dealing with fractions of countable items, such as the average number of cars per household.
Floating-point division by zero
Dividing a nonzero floating-point number by zero is undefined in regular arithmetic. Many programming languages produce an error when performing floating-point division by 0, but Java does not. Java handles this operation by producing infinity or -infinity, depending on the signs of the operands. Printing a floating-point variable that holds infinity or -infinity outputs Infinity or -Infinity.
If the dividend and divisor in floating-point division are both 0, the division results in a "not a number". Not a number (NaN) indicates an unrepresentable or undefined value. Printing a floating-point variable that is not a number outputs NaN.
Manipulating floating-point output
Some floating-point numbers have many digits after the decimal point. Ex: Irrational numbers (Ex: 3.14159265359...) and repeating decimals (Ex: 4.33333333...) have an infinite number of digits after the decimal. By default, most programming languages output at least 5 digits after the decimal point. But for many simple programs, this level of detail is not necessary. A common approach is to output floating-point numbers with a specific number of digits after the decimal to reduce complexity or produce a certain numerical type (Ex: Representing currency with two digits after the decimal). The syntax for outputting the double myFloat with two digits after the decimal point is
System.out.printf("%.2f", myFloat);
When outputting a certain number of digits after the decimal using printf(), Java rounds the last output digit, but the floating-point value remains the same. Manipulating how numbers are output is discussed in detail elsewhere.
2.8 Scientific notation for floating-point literals
Scientific notation is useful for representing floating-point numbers that are much greater than or much less than 0, such as 6.02 x 1023. A floating-point literal using scientific notation is written using an e preceding the power-of-10 exponent, as in 6.02e23 to represent 6.02 x 1023. The e stands for exponent. Likewise, 0.001 is 1 x 10-3 and can be written as 1.0e-3. For a floating-point literal, good practice is to make the leading digit non-zero.
2.9 Constant variables
A good practice is to minimize the use of literal numbers in code. One reason is to improve code readability. newPrice = origPrice - 5 is less clear than newPrice = origPrice - priceDiscount. When a variable represents a literal, the variable's value should not be changed in the code. If the programmer precedes the variable declaration with the keyword final, then the compiler will report an error if a later statement tries to change that variable's value. An initialized variable whose value cannot change is called a constant variable. A constant variable is also known as a final variable. A common convention, or good practice, is to name constant variables using upper case letters with words separated by underscores, to make constant variables clearly visible in code.
2.10 Using math methods
Basics
Some programs require math operations beyond +, -, *, /, like computing a square root. A standard Math class has about 30 math operations, known as methods. The Math class is part of Java's standard language package, so no import is required.
A method is a list of statements executed by invoking the method's name, such invoking known as a method call. Any method input values, or arguments, appear within ( ), separated by commas if more than one. A programmer must precede the method name with Math. to call a Math class method. Below, the method Math.sqrt() is called with one argument, areaSquare. The method call evaluates to a value, as in Math.sqrt(areaSquare) below evaluating to 7.0, with which sideSquare is assigned.
전달인자(Argument)는 메소드 호출시에 전달되는 값
매개변수(Parameter)는 메소드에서 전달 받은 값
Table 2.10.1: A few common methods from the standard Math class.
Method | Behavior | Example |
sqrt(x) | Square root of x | Math.sqrt(9.0) evaluates to 3.0. |
pow(x, y) | Power: xy | Math.pow(6.0, 2.0) evaluates to 36.0. |
abs(x) | Absolute value of x | Math.abs(-99.5) evaluates to 99.5. |
if you want to see more math method, refer this website Math (Java SE 12 & JDK 12 ) (oracle.com)
Math (Java SE 12 & JDK 12 )
Returns the fused multiply add of the three arguments; that is, returns the exact product of the first two arguments summed with the third argument and then rounded once to the nearest float. The rounding is done using the round to nearest even rounding mo
docs.oracle.com
Calls in arguments
Commonly a method call's argument itself includes a method call. Below, xy is computed via Math.pow(x, y). The result is used in an expression that is an argument to another call, in this case to Math.pow() again: Math.pow(2.0, Math.pow(x, y) + 1).
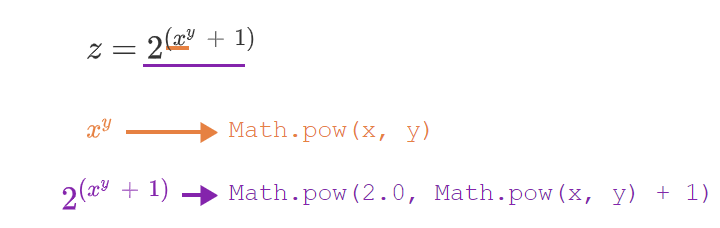
2.11 Integer division and modulo
Division: Integer rounding
When the operands of / are integers, the operator performs integer division, which does not generate any fraction.
The / operator performs floating-point division if at least one operand is a floating-point type.
Modulo (%)
The basic arithmetic operators include not just +, -, *, /, but also %. The modulo operator (%) evaluates the remainder of the division of two integer operands. Ex: 23 % 10 is 3.
Examples:
- 24 % 10 is 4. Reason: 24 / 10 is 2 with remainder 4.
- 50 % 50 is 0. Reason: 50 / 50 is 1 with remainder 0.
- 1 % 2 is 1. Reason: 1 / 2 is 0 with remainder 1.
Modulo examples
Modulo has several useful applications. Below are just a few.
Example 2.11.1: Random number in range.
Given a random number randNum, % can generate a random number within a range:
- randNum % 10
Yields 0 - 9: Possible remainders are 0, 1, ..., 8, 9. Remainder 10 is not possible: Ex: 19 % 10 is 9, but 20 % 10 is 0. - randNum % 51
Yields 0 - 50: Note that % 50 would yield 0 - 49. - (randNum % 9) + 1
Yields 1 - 9: The % 9 yields 9 possible values 0 - 8, so the + 1 yields 1 - 9. - (randNum % 11) + 20
Yields 20 - 30: The % 11 yields 11 possible values 0 - 10, so the + 20 yields 20 - 30.Example 2.11.3: Get prefix of a phone number.Given a 10-digit phone number stored as an integer, % and / can be used to get any part, such as the prefix. For phoneNum = 1365551212 (whose prefix is 555):
tmpVal = phoneNum / 10000; // / 10000 shifts right by 4, so 136555. prefixNum = tmpVal % 1000; // % 1000 gets the right 3 digits, so 555.
Dividing by a power of 10 shifts a value right. 321 / 10 is 32. 321 / 100 is 3.
% by a power of 10 gets the rightmost digits. 321 % 10 is 1. 321 % 100 is 21.
2.12 Type conversions
Type conversions
A calculation sometimes must mix integer and floating-point numbers. For example, given that about 50.4% of human births are males, then 0.504 * numBirths calculates the number of expected males in numBirths births. If numBirths is an int variable (int because the number of births is countable), then the expression combines a floating-point and integer.
A type conversion is a conversion of one data type to another, such as an int to a double. The compiler automatically performs several common conversions between int and double types, such automatic conversion known as implicit conversion.
- For an arithmetic operator like + or *, if either operand is a double, the other is automatically converted to double, and then a floating-point operation is performed.
- For assignments, the right side type is converted to the left side type if the conversion is possible without loss of precision.
int-to-double conversion is straightforward: 25 becomes 25.0.
double-to-int conversion may lose precision, so is not automatic.
Assigning doubles with integer literalsBecause of implicit conversion, statements like double someDoubleVar = 0; or someDoubleVar = 5; are allowed, but discouraged. Using 0.0 or 5.0 is preferable.Type casting
A programmer sometimes needs to explicitly convert an item's type. Ex: If a program needs a floating-point result from dividing two integers, then at least one of the integers needs to be converted to double so floating-point division is performed. Otherwise, integer division is performed, evaluating to only the quotient and ignoring the remainder. A type cast explicitly converts a value of one type to another type.
A programmer can precede an expression with (type) to convert the expression's value to the indicated type. Ex: If myIntVar is 7, then (double)myIntVar converts int 7 to double 7.0.
A common type cast converts a double to an int. Ex: myInt = (int)myDouble. The fractional part is truncated. Ex: 9.5 becomes 9.
The program below casts the numerator and denominator each to double so floating-point division is performed (actually, converting only one would have worked).
2.13 Binary
Normally, a programmer can think in terms of base ten numbers. However, a compiler must allocate some finite quantity of bits (e.g., 32 bits) for a variable, and that quantity of bits limits the range of numbers that the variable can represent. Thus, some background on how the quantity of bits influences a variable's number range is helpful.
Because each memory location is composed of bits (0s and 1s), a processor stores a number using base 2, known as a binary number.
For a number in the more familiar base 10, known as a decimal number, each digit must be 0-9 and each digit's place is weighed by increasing powers of 10.
2.14 Characters
Basics
A variable of char type, as in char myChar;, can store a single character like the letter m. A character literal is surrounded with single quotes, as in myChar = 'm';.
Getting a character from input
Java does not have a method for getting one character from input. Instead, the following sequence can be used: myChar = scnr.next().charAt(0); The charAt(0) is explained in another section. Briefly, next() gets the next sequence of non-whitespace characters (as a string), and charAt(0) gets the first character in that string.
A character is internally stored as a number
Under the hood, a char variable stores a number. Ex: 'a' is stored as 97. In an output statement, the compiler outputs the number's corresponding character.
ASCII is an early standard for encoding characters as numbers. The following table shows the ASCII encoding as a decimal number (Dec) for common printable characters (for readers who have studied binary numbers, the table shows the binary encoding also). Other characters such as control characters (e.g., a "line feed" character) or extended characters (e.g., the letter "n" with a tilde above it as used in Spanish) are not shown. Source: http://www.asciitable.com/.
Many earlier programming languages like C or C++ use ASCII. Java uses a more recent standard called Unicode. ASCII can represent 255 items, whereas Unicode can represent over 64,000 items; Unicode can represent characters from many different human languages, many symbols, and more. (For those who have studied binary: ASCII uses 8 bits, while Unicode uses 16, hence the 255 versus 64,000). Unicode's first several hundred items are the same as ASCII. The Unicode encoding for these characters has 0's on the left to yield 16 bits.
Outputting multiple character variables with one output statement
A programmer can output multiple character variables with one statement as follows: System.out.print("" + c1 + c2);. The initial "" tells the compiler to output a string of characters, and the +'s combine the subsequent characters into such a string. Without the "", the compiler will simply add the numerical values of c1 and c2, and output the resulting sum.
'Java' 카테고리의 다른 글
Chapter 03 Operator (0) | 2022.02.09 |
---|